Sample Java Script that displays a movable clock
By: Rajan in Java Tutorials on 2006-12-11
This code creates a movable clock using HTML5 Canvas and JavaScript. The clock has hour, minute, and second hands that move to display the current time. The clock can also be moved by clicking and dragging on the canvas.
The HTML code creates a canvas element with an id of "canvas" and includes a JavaScript file named "movable-clock.js". The canvas is styled to be positioned in the center of the page with a black border.
To test it, create two files in the same folder. First create movable-clock.html with the below code.
<!DOCTYPE html> <html> <head> <title>Movable Clock</title> <style> #canvas { position: absolute; left: 50%; top: 50%; transform: translate(-50%, -50%); border: 1px solid black; } </style> </head> <body> <canvas id="canvas" width="400" height="400"></canvas> <script src="movable-clock.js"></script> </body> </html>
Then create another file movable-clock.js in the same folder and copy and paste the below code.
// get the canvas element var canvas = document.getElementById("canvas"); // get the initial position of the canvas var initialX = 0; var initialY = 0; // add event listener for mouse down event canvas.addEventListener("mousedown", function(e) { // set the initial position of the canvas initialX = e.clientX; initialY = e.clientY; // add event listener for mouse move event document.addEventListener("mousemove", movecanvas); }); // add event listener for mouse up event document.addEventListener("mouseup", function() { // remove event listener for mouse move event document.removeEventListener("mousemove", movecanvas); }); // function to move the canvas function movecanvas(e) { // calculate the new position of the canvas var newX = initialX - e.clientX; var newY = initialY - e.clientY; // set the new position of the canvas canvas.style.left = canvas.offsetLeft - newX + "px"; canvas.style.top = canvas.offsetTop - newY + "px"; // set the new initial position of the canvas initialX = e.clientX; initialY = e.clientY; } // get the canvas context var canvas = document.getElementById("canvas"); var ctx = canvas.getContext("2d"); function drawcanvas() { // clear canvas ctx.clearRect(0, 0, canvas.width, canvas.height); var centerX = canvas.width / 2; var centerY = canvas.height / 2; var radius = canvas.width / 2 - 10; // draw canvas face ctx.beginPath(); ctx.arc(centerX, centerY, radius, 0, 2 * Math.PI); ctx.strokeStyle = "black"; ctx.lineWidth = 2; ctx.stroke(); // draw marks for (var i = 0; i < 12; i++) { var angle = i * (Math.PI / 6); var x = centerX + Math.sin(angle) * (radius - 10); var y = centerY - Math.cos(angle) * (radius - 10); ctx.beginPath(); ctx.arc(x, y, 5, 0, 2 * Math.PI); ctx.fillStyle = "black"; ctx.fill(); } // draw hands var d = new Date(); var hour = d.getHours(); var minute = d.getMinutes(); var second = d.getSeconds(); var hourAngle = (hour % 12) * (Math.PI / 6) + (minute / 60) * (Math.PI / 6) + (second / 3600) * (Math.PI / 6); var minuteAngle = minute * (Math.PI / 30) + (second / 1800) * (Math.PI / 30); var secondAngle = second * (Math.PI / 30); // draw hour hand ctx.beginPath(); ctx.moveTo(centerX, centerY); ctx.lineTo(centerX + Math.sin(hourAngle) * (radius - 60), centerY - Math.cos(hourAngle) * (radius - 60)); ctx.strokeStyle = "black"; ctx.lineWidth = 8; ctx.lineCap = "round"; ctx.stroke(); // draw minute hand ctx.beginPath(); ctx.moveTo(centerX, centerY); ctx.lineTo(centerX + Math.sin(minuteAngle) * (radius - 40), centerY - Math.cos(minuteAngle) * (radius - 40)); ctx.strokeStyle = "black"; ctx.lineWidth = 4; ctx.lineCap = "round"; ctx.stroke(); // draw second hand ctx.beginPath(); ctx.moveTo(centerX, centerY); ctx.lineTo(centerX + Math.sin(secondAngle) * (radius - 20), centerY - Math.cos(secondAngle) * (radius - 20)); ctx.strokeStyle = "red"; ctx.lineWidth = 2; ctx.lineCap = "round"; ctx.stroke(); // request animation frame requestAnimationFrame(drawcanvas); } // draw the canvas every second setInterval(drawcanvas, 1000);
Now open the movable-clock.html in your browser and you should see something like below.
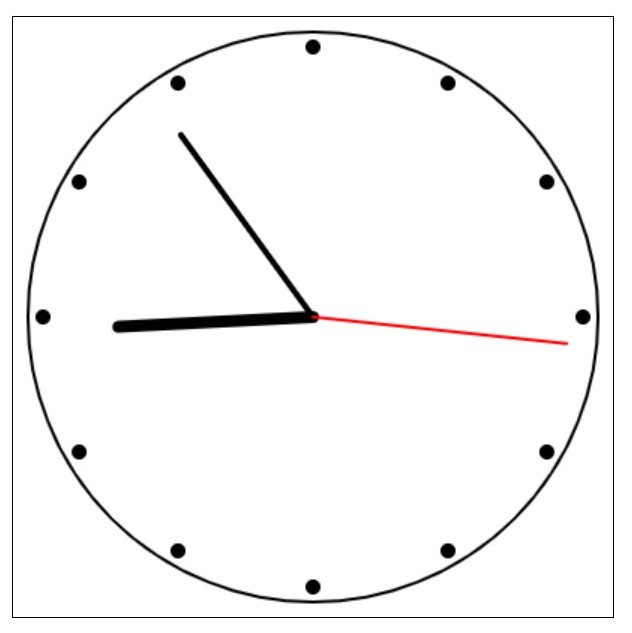
Add Comment
This policy contains information about your privacy. By posting, you are declaring that you understand this policy:
- Your name, rating, website address, town, country, state and comment will be publicly displayed if entered.
- Aside from the data entered into these form fields, other stored data about your comment will include:
- Your IP address (not displayed)
- The time/date of your submission (displayed)
- Your email address will not be shared. It is collected for only two reasons:
- Administrative purposes, should a need to contact you arise.
- To inform you of new comments, should you subscribe to receive notifications.
- A cookie may be set on your computer. This is used to remember your inputs. It will expire by itself.
This policy is subject to change at any time and without notice.
These terms and conditions contain rules about posting comments. By submitting a comment, you are declaring that you agree with these rules:
- Although the administrator will attempt to moderate comments, it is impossible for every comment to have been moderated at any given time.
- You acknowledge that all comments express the views and opinions of the original author and not those of the administrator.
- You agree not to post any material which is knowingly false, obscene, hateful, threatening, harassing or invasive of a person's privacy.
- The administrator has the right to edit, move or remove any comment for any reason and without notice.
Failure to comply with these rules may result in being banned from submitting further comments.
These terms and conditions are subject to change at any time and without notice.
- Data Science
- Android
- React Native
- AJAX
- ASP.net
- C
- C++
- C#
- Cocoa
- Cloud Computing
- HTML5
- Java
- Javascript
- JSF
- JSP
- J2ME
- Java Beans
- EJB
- JDBC
- Linux
- Mac OS X
- iPhone
- MySQL
- Office 365
- Perl
- PHP
- Python
- Ruby
- VB.net
- Hibernate
- Struts
- SAP
- Trends
- Tech Reviews
- WebServices
- XML
- Certification
- Interview
categories
Related Tutorials
Read a file having a list of telnet commands and execute them one by one using Java
Open a .docx file and show content in a TextArea using Java
Step by Step guide to setup freetts for Java
Of Object, equals (), == and hashCode ()
Using the AWS SDK for Java in Eclipse
DateFormat sample program in Java
concurrent.Flow instead of Observable class in Java
Calculator application in Java
Sending Email from Java application (using gmail)
Comments